引言
壹、Feign的简介
Feign是一个声明式的伪Http客户端,它使得写Http客户端变得更简单。使用Feign,只需要创建一个接口并注解。它具有可插拔的注解特性,可使用Feign 注解和JAX-RS注解。Feign支持可插拔的编码器和解码器。Feign默认集成了Ribbon,并和Eureka结合,默认实现了负载均衡的效果。
简而言之:
- Feign 采用的是基于接口的注解
- Feign 整合了ribbon
贰、准备工作
新建一个feign子工程lovin-feign-client,用于后面的操作。下面是主要的pom依赖:~~~pomlovincloudcom.eelve.lovincloud1.0-SNAPSHOT4.0.0
<artifactId>lovin-feign-client</artifactId> <version>0.0.1</version> <name>lovinfeignclient</name> <description>feignclient测试</description>复制代码 <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-hystrix</artifactId> <version>1.4.7.RELEASE</version> </dependency> </dependencies>复制代码 <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> ~~~ - 这里为了安全,我这里还是添加**spring-boot-starter-security** ~~~yaml server: port: 8806 # 服务端口号 spring: application: name: lovinfeignclient # 服务名称 security: basic: enabled: true user: name: lovin password: ${REGISTRY_SERVER_PASSWORD:lovin} eureka: client: serviceUrl: defaultZone: http://lovin:lovin@localhost:8881/eureka/ # 注册到的eureka服务地址 feign: hystrix: enabled: true ~~~ - 配置**spring-boot-starter-security**,这里为了方便我这里放开所有请求 ~~~java package com.eelve.lovin.config;复制代码
import org.springframework.context.annotation.Configuration;import org.springframework.security.config.annotation.web.builders.HttpSecurity;import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
/**
- @ClassName SecurityConfig
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/16 14:13
- @Version 1.0
- **/
- @Configuration
- public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests().anyRequest().permitAll() .and().csrf().disable(); } } ~~~ - 在主类上添加**@EnableFeignClients**和**@EnableHystrix** ,当然也需要注册到注册中心: ~~~java package com.eelve.lovin;复制代码
import org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;import org.springframework.cloud.client.discovery.EnableDiscoveryClient;import org.springframework.cloud.netflix.hystrix.EnableHystrix;import org.springframework.cloud.openfeign.EnableFeignClients;
/**
- @ClassName LovinFeignClientApplication
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/15 17:17
- @Version 1.0
- **/
- @SpringBootApplication
- @EnableFeignClients
- @EnableDiscoveryClient
- @EnableHystrix
- public class LovinFeignClientApplication {
- public static void main(String[] args) {
- SpringApplication.run(LovinFeignClientApplication.class,args);
- }
- }
- ~~~
- 添加一个远程调用的服务端FeignRemoteService,并且配置feign调用信息:
- ~~~java
- package com.eelve.lovin.service;
import com.eelve.lovin.hystrix.FeignRemoteServiceImpl;import org.springframework.cloud.openfeign.FeignClient;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RequestMethod;
/**
- @ClassName FeignRemoteService
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/15 17:18
- @Version 1.0
- **/
- @FeignClient(value = "lovineurkaclient",fallback = FeignRemoteServiceImpl.class)
- public interface FeignRemoteService {
@RequestMapping(value = "/hello",method = RequestMethod.GET) public String hello(); } ~~~ - 添加熔断器调用方法:新建**FeignRemoteServiceImpl**实现**FeignRemoteService**接口: ~~~java package com.eelve.lovin.hystrix;复制代码
import com.eelve.lovin.service.FeignRemoteService;import org.springframework.stereotype.Component;
/**
- @ClassName FeignRemoteServiceImpl
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/15 17:31
- @Version 1.0
- **/
- @Component
- public class FeignRemoteServiceImpl implements FeignRemoteService {
- @Override
- public String hello() {
- return "hystrix起作用了";
- }
- }
- ~~~
- 最后新建FeignController,来消费服务:
- ~~~java
- package com.eelve.lovin.controller;
import com.eelve.lovin.service.FeignRemoteService;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestParam;import org.springframework.web.bind.annotation.RestController;
/**
- @ClassName FeignController
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/15 17:21
- @Version 1.0
- **/
- @RestController
- public class FeignController {
@Autowired FeignRemoteService feignRemoteService;复制代码 @GetMapping(value = "/getHello") public String getHello() { return feignRemoteService.hello(); } } ~~~ # 叁、启动测试 - 依次启动eureka的服务端和两个客户端,以及新建的lovin-feign-client 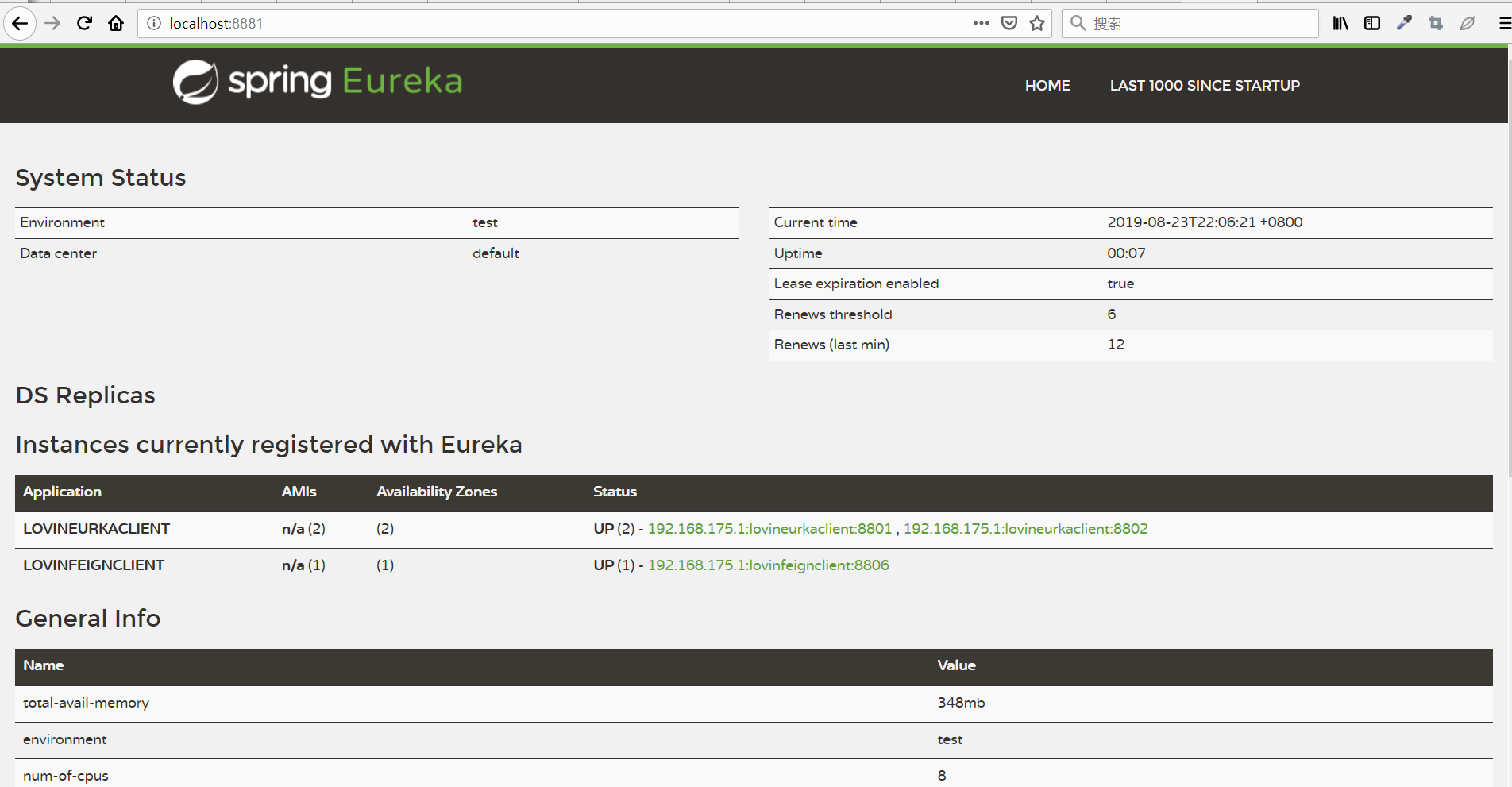 我们可以看到服务已经全部启动成功 - 然后访问http://localhost:8806/getHello  我们可以看到已经可以通过feign调到我们建立的eureka客户端了 - 再次请求接口观察返回  我们可以看到我们调到了通过feign调用ribbon负载的另外一个接口了,到这里我们就已经弄好了一个简单的ribbon负载。复制代码
肆、添加Hystrix Dashboard断路器监控
- 添加需要的pom依赖
- ~~~pom
- org.springframework.boot
- spring-boot-starter-actuator
- org.springframework.cloud
- spring-cloud-starter-netflix-hystrix-dashboard
- 2.1.2.RELEASE
- ~~~
- 在主类上添加@EnableHystrixDashboard,开启断路器监控,并且配置HystrixMetricsStreamServlet
- ~~~java
- package com.eelve.lovin;
import com.netflix.hystrix.contrib.metrics.eventstream.HystrixMetricsStreamServlet;import org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;import org.springframework.boot.web.servlet.ServletRegistrationBean;import org.springframework.cloud.client.discovery.EnableDiscoveryClient;import org.springframework.cloud.netflix.hystrix.EnableHystrix;import org.springframework.cloud.netflix.hystrix.dashboard.EnableHystrixDashboard;import org.springframework.cloud.openfeign.EnableFeignClients;import org.springframework.context.annotation.Bean;
/**
- @ClassName LovinFeignClientApplication
- @Description TDO
- @Author zhao.zhilue
- @Date 2019/8/15 17:17
- @Version 1.0
- **/
- @SpringBootApplication
- @EnableFeignClients
- @EnableDiscoveryClient
- @EnableHystrix
- @EnableHystrixDashboard
- public class LovinFeignClientApplication {
- public static void main(String[] args) {
- SpringApplication.run(LovinFeignClientApplication.class,args);
- }
@Bean public ServletRegistrationBean getServlet(){ HystrixMetricsStreamServlet streamServlet = new HystrixMetricsStreamServlet(); ServletRegistrationBean registrationBean = new ServletRegistrationBean(streamServlet); registrationBean.setLoadOnStartup(1); registrationBean.addUrlMappings("/actuator/hystrix.stream"); registrationBean.setName("HystrixMetricsStreamServlet"); return registrationBean; } } ~~~ - 访问http://localhost:8806/hystrix  这里我们通过首页可以看到: ~~~ 默认的集群监控:通过URL http://turbine-hostname:port/turbine.stream开启,实现对默认集群的监控。 指定的集群监控:通过URL http://turbine-hostname:port/turbine.stream?cluster=[clusterName]开启,实现对clusterName的监控。 单体应用监控:通过URL http://hystrix-app:port/hystrix.stream开启,实现对某个具体的服务监控 ~~~ - 添加监控模式查看详情,这里选择第三个单体应用复制代码
这样我们就完成了熔断器的监控,当然具体含义有待下一步深究。
伍、网络架构
- 我们可以看到我们调用的服务不再是像再上一篇文章中的直接访问对应的服务,而是通过feign的Ribbon的负载均衡的去调用的,而且这里说明一点,Ribbon的默认机制是轮询。
小结
欢迎关注头条号:JAVA大飞哥
点击关注评论转发一波:私信小编发送“架构”(免费获取)
获取微服务、分布式、高并发、高可用,性能优化丶Mysql源码分析等等一些技术资料
最后,每一位读到这里的Java程序猿朋友们,感谢你们能耐心地看完。希望在成为一名更优秀的Java程序猿的道路上,我们可以一起学习、一起进步!都能赢取白富美,走向架构师的人生巅峰!
本文暂时没有评论,来添加一个吧(●'◡'●)